React Native has become a cornerstone in cross-platform app development, offering flexibility and speed to developers aiming for a native-like experience on diverse devices. Despite its popularity, React Native encounters performance challenges, such as animation handling and limitations in multitasking capabilities. The community’s continuous efforts to enhance React Native are commendable, but incorporating new features sometimes leads to performance bottlenecks.
This article delves into the reasons behind slow React Native app performance and provides an extensive guide on optimizing and boosting the overall efficiency of your applications.
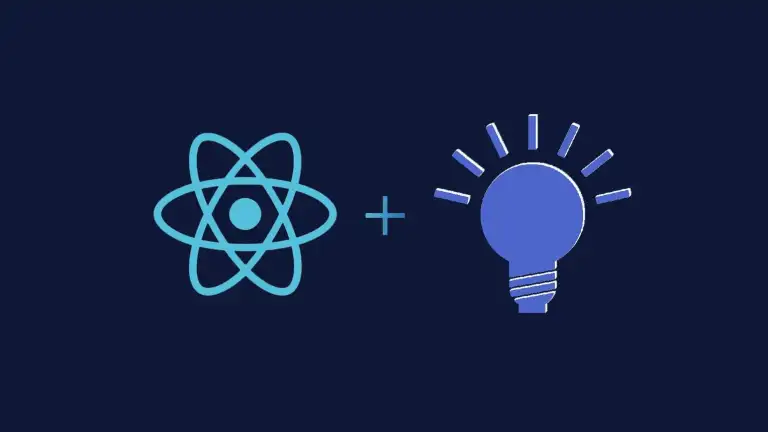
Reasons for Slow React Native App Performance
Understanding the factors contributing to slow performance is crucial before delving into optimization techniques. Several common reasons can hinder the speed of React Native apps:
- Complex UI: Apps with intricate UI designs, involving numerous nested components, may experience slower rendering times, leading to performance issues.
- Complex Animations: Poorly implemented or overly intricate animations can significantly slow down component rendering, impacting the overall user experience.
- Unnecessary Re-renders:Overusing setState or neglecting the shouldComponentUpdate lifecycle method can result in unnecessary re-renders, leading to performance overhead.
- Optimization Shortcomings: Inadequate optimization practices, such as using inappropriate components or overlooking performance optimization techniques like memoization, can hamper app speed.
- Complex Calculations: Performing intricate calculations within components can delay rendering and impact overall performance.
- Data Fetching Impact: Fetching excessive data for a specific component or page can increase loading times and undermine overall performance.
Now that we’ve identified these potential pitfalls, let’s explore strategies to improve React Native app performance.
Ways to Improve React Native App Performance
Network Optimization
Network operations can significantly influence app performance. To optimize network usage, consider the following:
- Consolidate API calls or adopt more efficient data retrieval approaches to minimize the number of HTTP requests.
- Implement caching systems to reduce network round-trips, especially for static or less frequently updated data.
- Leverage libraries like ‘react-native-offline’ or ‘react-native-fast-image’ to enable offline caching and optimize image loading for improved efficiency.
Optimize Launch Speed
The speed at which an app is launched contributes to its overall perceived performance. To enhance launch speed:
- Optimize rendering to ensure smooth launches by reducing memory usage and minimizing bundle size.
- Leverage React Native features like Hermes integration and Memoization to improve rendering efficiency.
Improve Debugging Speed
Efficient debugging is essential for maintaining productivity and app speed. Use tools like Flipper, which integrates well with React Native and native app systems. It provides a comprehensive layout, including a network inspector and a detailed log, facilitating efficient debugging.
Cache Image Files
Caching image files is crucial for resolving issues related to loading and re-rendering images from remote endpoints:
- Implement manual caching by downloading images to local storage in the app’s cache directory, improving loading efficiency.
- Consider using the ‘react-native-fast-image’ library, which seamlessly handles image caching, enhancing overall image loading performance.
import FastImage from 'react-native-fast-image'
const App = () => (
<FastImage
style={{ ... }}
source={{
uri: 'https://unsplash.it/200/200?image=8',
priority: FastImage.priority.normal,
cache: FastImage.cacheControl.cacheOnly
}}
/>
)
Optimize Images
Optimizing images is crucial for preventing delays during app launch:
- Use SVG for small icons and PNG for detailed images like product photos.
- Employ lazy-loading techniques for images to defer loading until necessary, minimizing unnecessary network requests.
- Avoid excessively high resolutions for images.
- Utilize the WEBP format to reduce image size for both iOS and Android platforms.
Optimize Rendering
Efficient rendering is essential for enhancing app performance:
- Use the ‘VirtualizedList’ component for efficient rendering of large lists.
- Minimize unnecessary re-renders by using ‘PureComponent’ or ‘React.memo’ for components.
- Opt for the Animated API over inline styles to ensure smoother animations.
Memory Management
Effective memory management is pivotal for app performance:
- Unsubscribe from event listeners, clear intervals, and eliminate object references when no longer required to prevent memory leaks.
- Limit the usage of the React Native bridge and minimize unnecessary data conversions between JavaScript and native code.
- Employ efficient data structures and algorithms to decrease memory usage and enhance overall performance.
Remove Unnecessary Libraries and Features
Every additional library contributes to the app’s size and impact. Selectively incorporate only necessary libraries and functionalities:
- Include only essential features to avoid unnecessary dependencies that can impact screen load times.
Optimize React Native Code
Optimizing code is crucial for managing time and memory resources effectively:
- Use ‘PureComponent’ for text elements to prevent unnecessary overrides and potential performance impacts.
- Define functions and code accurately, and introduce the most suitable code for each scenario.
Profile Your App
Profiling your React Native app is crucial for pinpointing and improving performance issues. Profiling tools such as React Native Performance, Flipper, or Chrome DevTools can be employed to analyze CPU usage, rendering speed, and memory consumption. Focus on optimizing functions and components with high execution times, address slow rendering through component optimization, and identify and fix memory leaks. This targeted approach enhances your app’s responsiveness and overall user experience.
Conclusion
In conclusion, ensuring a smooth and responsive user experience in your React Native app is achievable through effective performance optimization. By employing the outlined strategies, developers can refine rendering, minimize app size, adeptly handle memory, optimize code, and streamline network operations. Consistently profiling your app helps identify and address fresh performance issues, allowing for continuous refinement.
A finely tuned React Native app not only meets user expectations but also contributes to the overall success of your application. As mobile users increasingly demand faster and more efficient experiences, developers must prioritize performance optimization as an integral part of the app development lifecycle.
By following these strategies and embracing a proactive approach to performance optimization, developers can create React Native apps that stand out for their speed, efficiency, and delightful user experiences.
Tags: React, React Native