React JS has firmly established itself as a go-to JavaScript library for developing dynamic and user-friendly interfaces. Renowned for its simplicity, flexibility, and commendable performance, React JS introduces developers to two pivotal concepts: state and props. In this comprehensive guide, we’ll delve into the intricacies of these concepts, unraveling their significance in React JS development.
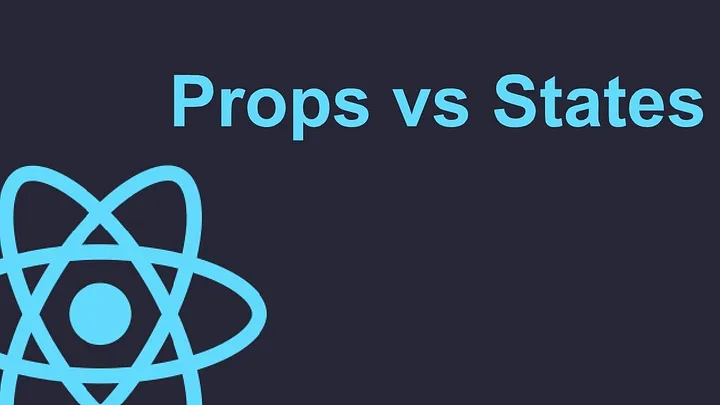
Understanding State in React JS
What is State?
In the realm of React JS, state is a fundamental object that empowers components to store and manage their internal data. Serving as a repository for a component’s current state, it is initialized in the constructor method and can be dynamically updated using the ‘setState()’ method. Crucially, any alteration in a component’s state triggers an automatic re-rendering, ensuring that the UI accurately reflects the modified state.
How Does State Work?
State operates as a mechanism enabling components to independently manage their data. When a component’s state undergoes a transformation, React responds by triggering a re-render, facilitating the creation of dynamic and interactive user interfaces. This intrinsic feature simplifies the development of applications with real-time responsiveness.
How to Use State in Your Code
To harness the power of state in your React components, the initialization process begins in the constructor method:
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
handleClick() {
this.setState({
count: this.state.count + 1
});
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={() => this.handleClick()}>
Click me
</button>
</div>
);
}
}
In this example, the state of the component is initialized with a single property, ‘count’. The ‘handleClick’ method, invoked when the button is clicked, utilizes ‘setState()’ to increment the ‘count’ property. This dynamic approach to managing state facilitates the creation of responsive interfaces.
Examples of State in Action
- Toggle a Button: Leverage state to toggle the button’s state between “on” and “off” upon a click event.
- Display a Counter: Utilize state to keep track of the number of button clicks and dynamically display the count to the user.
- Show or Hide Content: Leverage state to conditionally render content based on user interaction, providing a seamless and interactive user experience.
Understanding Props in React JS
What are Props?
Props, short for properties, are read-only values that facilitate the transfer of data across React components. Comparable to function arguments in JavaScript or attributes in HTML, props play a crucial role in creating dynamic and data-driven React applications.
How Do You Pass Data with Props?
Passing data with props involves utilizing a syntax akin to HTML attributes. For instance, an object and a number can be passed as props to a child component:
<ChildComponent person={{ name: 'John Doe', age: 30 }} count={10} />
In this example, an object with properties (‘name’ and ‘age’) and a number (‘count’) is passed as props to the ‘ChildComponent’.
Examples of Props in Action
- Displaying Data: Use props to display data in React components, such as passing an object with user information to a ‘UserProfile’ component to display the user’s name, age, and profile picture.
- Passing Functions: Pass functions as props to enable child components to communicate with their parent components. For instance, passing a function that updates the state of a parent component to a child component, calling it when a button is clicked.
- Conditional Rendering: Leverage props for conditional rendering in React components. For example, pass a boolean value as props to a ‘ShowHide’ component and render different content based on whether the value is true or false.
Differences Between State and Props
Despite being fundamental to React JS, state and props exhibit key differences:
- Ownership: State is owned and managed within the component itself, while props are owned by the parent component and passed down to child components.
- Mutability: State is mutable and can be changed using the ‘setState()’ method, while props are immutable and cannot be modified by the child component.
- Access: State is local to the component and can only be accessed and modified within that component. In contrast, props can be accessed by the child component but cannot be modified.
- Usage: State is utilized to manage data local to the component, whereas props are employed to pass data between components.
State and Props in Harmony
In the symphony of React JS development, state and props harmonize to orchestrate seamless and interactive user experiences. State empowers components to manage their internal data dynamically, enabling responsiveness to user interactions. On the other hand, props facilitate the flow of data across components, fostering consistency and enabling the creation of modular and reusable code.
Best Practices for State and Props Management
Keep State Local: Whenever possible, keep state local to the component that truly needs it. Avoid unnecessary global state management for simpler components.
Props for Communication: Leverage props for communication between parent and child components. This promotes a unidirectional data flow and simplifies the understanding of data sources.
Immutability Matters: When updating state using ‘setState()’, ensure immutability. Create a new object or array with the updated values instead of modifying the existing state directly.
// Incorrect
this.state.items.push(newItem);
// Correct
this.setState({
items: [...this.state.items, newItem]
});
Use Functional setState: When the new state depends on the current state, use the functional form of ‘setState’ to avoid race conditions.
// Without functional setState
this.setState({
count: this.state.count + 1
});
// With functional setState
this.setState((prevState) => ({
count: prevState.count + 1
}));
Destructure Props: When accessing multiple props in a component, consider destructuring them for cleaner and more readable code.
// Without destructuring
const Article = (props) => {
return <h2>{props.title}</h2>;
// With destructuring
const Article = ({ title }) => {
return <h2>{title}</h2>;
Conclusion
In the grand tapestry of React JS development, state and props emerge as fundamental threads that weave together dynamic, responsive, and modular applications. State empowers components to manage their internal data dynamically, fostering interactive user experiences. In contrast, props facilitate seamless communication between components, enhancing reusability and maintainability.
By adhering to best practices, embracing the innate characteristics of state and props, and exploring their diverse applications, developers can navigate the React JS landscape with confidence. As the React ecosystem evolves, the mastery of state and props remains a cornerstone in the pursuit of creating exceptional and user-centric applications.
Tags: React, ReactJS, State and Prop