React 18 was released in March 2022. Officially, React 18 is now ready to use! This release focuses on performance improvements and updating the rendering engine. React 18 sets the foundation for concurrent rendering APIs that future React features will be built on top of.
In my opinion, the latest feature that revolutionized the React Ecosystem was React Hooks, introduced in React 16.8 back in 2019. Since then, we have seen many versions being released. But without any major changes, what will happen in React 18? In this tutorial, we will have a quick look at the features released in React 18, and explain a few major concepts such as concurrent rendering, automatic batching, and transitions.
New Features
Automatic Batching
React 18 features automatic batching. To understand batching, let’s consider the example of grocery shopping from the same React Working Group discussion.
Let’s say that you are making pasta for dinner. If you were to optimize your grocery trip, you would create a list of all the ingredients that you need to buy, make a trip to the grocery store, and get all your ingredients in one trip. This is batching.
Without batching, you would start cooking, find out you need an ingredient, go to the grocery store and buy the ingredient. When you come back and continue cooking, you’ll find out you need another ingredient, you’ll then go to the grocery store again to buy that ingredient
In React, batching helps to reduce the number of re-renders that happen when a state changes, when you call setState. With this new exciting feature, React will combine multiple setState() calls into a single re-render to improve performance. Before this, if we had multiple setState() inside of a setTimeout(), React will re-render for every state update which sometimes causes performance issues if we have larger components. Previously, React batched state updates in event handlers.
You may already be familiar with this. This feature was available in React 17, but now it comes out of the box.
// React 17
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// The component will be rendered twice.
}, 1000);
// React 18
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// The component will be rendered only once.
}, 1000);
Transitions
This new feature lets the dev split the state update into two categories: urgent and low priority.
Transitions can be used to mark UI updates that do not need urgent resources for updating. For some actions, like selecting in a dropdown or updating an input, you want the action to respond immediately, so now we could distinguish between an urgent update and a non-urgent one. These non-urgent updates are called transitions. By marking non-urgent UI updates as “transitions”, React will know which updates to prioritize. This makes it easier to optimize rendering and get rid of stale rendering.
Updates wrapped in startTransition are handled as non-urgent and will be interrupted if more urgent updates like clicks or keypresses come in.
import { startTransition } from 'react';
// Updating state with urgent priority
setInputValue(input);
// Inside startTransition the updates won't be urgent
// and can be interrupted
startTransition(() => {
setSearchQuery(input);
});
New Suspense Features
Finally, we will have Suspense on the server. In the server-rendered apps, we will have only one Suspense API.
In a client-rendered app, you load the HTML of your page from the server along with all the JavaScript that is needed to run the page and make it interactive.
If, however, the JavaScript bundle is huge, or you have a slow connection, this process can take a long time and the user will be waiting for the page to become interactive, or to see meaningful content. For optimizing the user experience and avoiding the user having to sit on a blank screen, we can use server rendering.
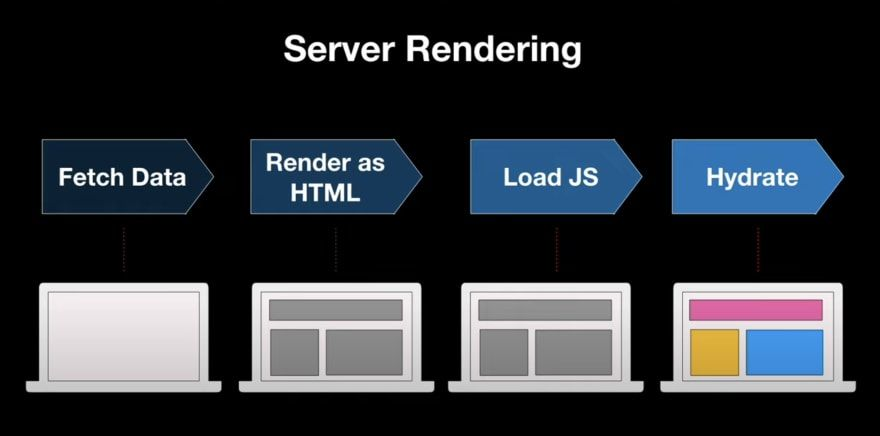
Server rendering is a technique where you render the HTML output of your React components on the server and send HTML from the server. This lets the user view some UI while JS bundles are loading and before the app becomes interactive. Server rendering further enhances the user experience of loading the page and reducing the time to interact.
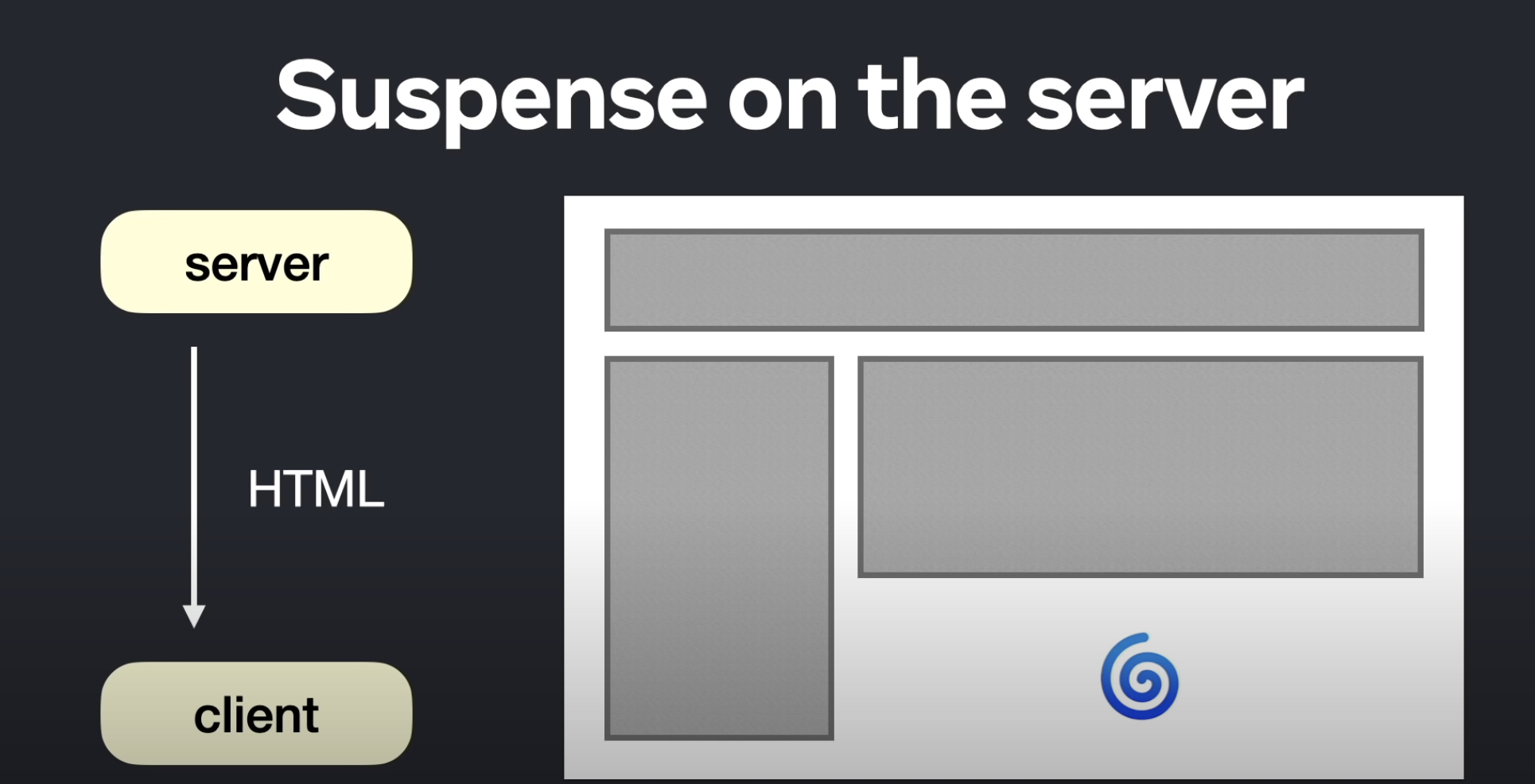
Before React 18, this part was often the bottleneck of the app and would increase the time it took to render the component. Now React 18 adds support for Suspense on the server. With the help of suspense, you can wrap a slow part of your app within the Suspense component, telling React to delay the loading of the slow component. This can also be used to specify a loading state that can be shown while it’s loading.
Strict mode
Strict mode in React 18 will simulate mounting, unmounting, and re-mounting the component with a previous state. This sets the ground for a reusable state in the future where React can immediately mount a previous screen by remounting trees using the same component state before unmounting.
Strict mode will ensure components are resilient to the effects of being mounted and unmounted multiple times.
IE11 no longer supported
IE11 is no longer supported since React 18. If your app needs to continue bringing support to IE11, you will need to stay at React 17. x.
Conclusion
Some cool features like Automatic Batching or Adding Suspense should improve the performance of React in the latest release. I’m really excited to see how these new features will perform in a real-life app, but they look more than promising. In a summary, React 18 sets the foundation for future releases and focuses on improving the user experience.
Upgrading to React 18 should be straightforward and your existing code should not break after the update. The upgrade process should not take more than an afternoon.
Article published on May 15, 2022
If you like this article, please share it: |
Tags: React 18