Dev Improve Your React App with These Custom Hooks Streamlining Development to Improve EfficiencyIn the ever-evolving landscape of web development, React has emerged as a cornerstone technology, empowering developers to build dynamic and interactive user interfaces with ease. With the introduction of React Hooks, developers gained a powerful toolset for managing state, side effects, and logic within functional components. However, the true potential of React Hooks extends beyond the built-in ones provided by the framework.
In this article, we’ll delve into the world of custom React Hooks – a vast ecosystem of reusable, composable functions that extend the capabilities of React and streamline development workflows. These custom hooks, crafted by developers worldwide, address common challenges, simplify complex tasks, and unlock new possibilities for React applications. Join us on a journey through 11 essential custom React Hooks, each offering insights, examples, and practical applications to elevate your React projects to new heights. Whether you’re a seasoned React developer or just getting started, this guide will equip you with the tools and knowledge needed to harness the full potential of React Hooks in your applications.
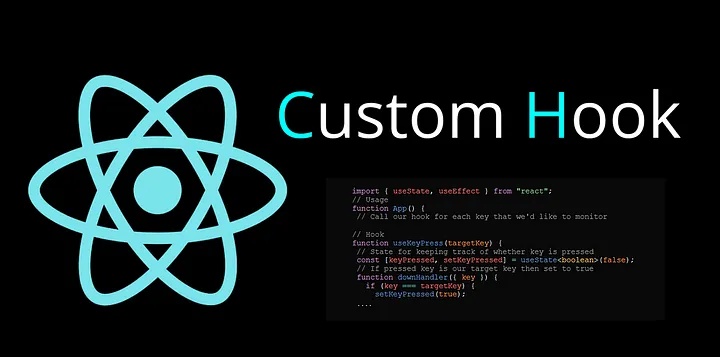
useScript
The ‘useScript’ hook in React simplifies the dynamic loading of external scripts. With just a few lines of code, developers can effortlessly integrate third-party dependencies into their applications.
Example:
import useScript from "react-script-hook"
function Component() {
const [ loading, error ] = useScript({
src: "analytics.google.com/api/v2/",
onload: () => console.log("Script loaded")
})
if(loading) return <div>Script loading</div>
if(error) return <div>Error occured</div>
return (
<div>
...
</div>
)
}
export default MyComponent;
export default MyComponent;
In this example, the ‘useScript’ hook loads an external script’. The load attribute shows when the foreign script has finished loading. We examine the loading and error flags and show warnings accordingly.
useLocalStorage
The useLocalStorage hook in React simplifies storing and accessing data in the browser’s localStorage. With a single line of code, it offers a clean API for managing persistent data. For instance:
For installing:
npm i @rehooks/local-storage
For importing:
const [name, setName] = useLocalStorage('username', 'Guest');
Here, ‘username’ is the key in localStorage, and ‘Guest’ is the default value. The hook returns the current value (‘name’) and a function (‘setName’) to update it. This makes handling local storage operations straightforward and concise.
import { useLocalStorage } from "@rehooks/local-storage"
function Component() {
const [ name, setName, deleteName ] = useLocalStorage("name")
return (
<div>
<div>Key: "name" | Value: "{name}"</div>
<div>
<button onClick={ ()=> setName("nnamdi")}>Set Name</button>
<button onClick={deleteName}>Delete Value</button>
</div>
</div>
)
}
use-mouse-action
The ‘use-mouse-action’ custom React hook is a valuable tool for handling mouse events within React components. With this hook, developers can easily listen to mouse actions such as clicks, mouse downs, and mouse ups, providing enhanced interactivity and user engagement.
This hook offers three distinct functions:
- useMouseAction: Registers mouse actions on a specific element, allowing developers to respond to various mouse events with custom logic.
- useMouseDown: Specifically captures mouse down events on an element, enabling targeted interactions and behaviors when the mouse button is pressed.
- useMouseUp: Captures mouse up events on an element, facilitating responsive feedback and actions when the mouse button is released.
To import:
import { useMouseAction, useMouseDown, useMouseUp } from "use-mouse-action"
For Example:
import { useMouseAction, useMouseDown, useMouseUp } from "use-mouse-action"
function Component() {
const mouseActionProps = useMouseAction({
onAction: () => console.log("Mouse clicked")
})
const mouseDownProps = useMouseDown(() => console.log("Mouse down"))
const mouseUpProps = useMouseUp(() => console.log("Mouse up"))
return (
<>
<button {...mouseActionProps}>Mouse Action</button>
<button {...mouseDownProps}>Mouse Down</button>
<button {...mouseUpProps}>Mouse Up</button>
</>
)
}
useSpeechRecognition
This React hook enables real-time speech-to-text conversion, leveraging the Web Speech API. Developers can integrate speech recognition functionality into their applications effortlessly. For instance:
import { useSpeechRecognition } from "react-speech-kit"
function Component() {
const [ result, setResult ] = useState()
const { listen, listening, stop } = useSpeechRecognition({
onResult: result => setResult(result)
})
return (
<div>
{listening ? "Speak, I'm listening" : ""}
<textarea value={value} />
<button onClick={listen}>Listen</button>
<button onClick={stop}>Stop</button>
</div>
)
}
This example demonstrates a simple component that starts and stops listening for speech input, providing immediate feedback to the user.
useNetworkStatus
The ‘useNetworkStatus’ hook is a valuable addition to any React application, providing real-time information about the user’s network connection. By accessing properties from the ‘navigator.connection’ object, this hook offers insights into the network environment, empowering developers to tailor their applications accordingly.
For example, consider a messaging application that allows users to send messages in real-time. By utilizing ‘useNetworkStatus’, the application can dynamically adjust its behavior based on the user’s network conditions. If the user has a slow connection, the application can prioritize sending text messages over media files to minimize latency. Conversely, when the user is on a fast connection, the application can optimize media file uploads for quicker delivery.
Here’s a simplified example of how to use ‘useNetworkStatus’:
import useNetworkStatus from "@rehooks/network-status"
function Component() {
const connection = useNetworkStatus()
const { effectiveType, saveData, rtt, downlink } = connection;
return (
<div>
<div>Network: {connection.effectiveType ? "Fast" : "Slow"}</div>
<div>Data Saver Mode: {saveData ? "Yes" : "No" }</div>
</div>
)
}
export default NetworkStatusComponent;
The above code simply displays the network status of the user using the ‘useNetworkStatus’ hook.
useDocumentTitle
This React hook enables seamless management of document titles, ensuring consistency between application state and browser tabs. By dynamically updating the title, developers can provide contextually relevant information to users, enhancing overall user experience.
Example:
import React from 'react';
import { useDocumentTitle } from '@rehooks/document-title';
const App = () => {
useDocumentTitle('My Awesome App');
return (
<div>
<h1>Welcome to My Awesome App!</h1>
{/* Other components and content */}
</div>
);
};
export default App;
In this example, the document title will be set to “My Awesome App” when the component mounts, ensuring that users see the appropriate title in their browser tabs.
Wrapping Up
These custom React Hooks are a game changer in modern web development, giving developers unprecedented flexibility and efficiency when creating dynamic user interfaces. By using the power of these hooks, developers may expedite typical operations, improve interaction, and open up new possibilities in React apps. From simplifying script integration with ‘useScript’ to enabling real-time voice recognition with ‘useSpeechRecognition’, the ecosystem of custom hooks is growing, allowing developers to push the boundaries of what is possible with React. Armed with this knowledge, developers are ready to take their projects to new heights, providing richer experiences and fostering innovation in the ever-changing web development industry.
Tags: Development, React, Visual Studio